Introduction
There's a good example on how to use jQueryUI slider contolls to choose a color in the jQueryUI's webpage.
Today we'll take that one step further and create a color chooser dialog widget for use in websites.
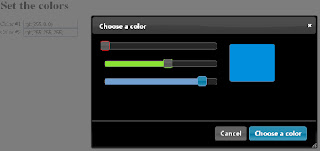
Step 1 - Get the libraries
Start off by downloading the jQueryUI library from http://www.jqueryui.com with a theme of your choosing.
I downloaded the whole library, but not all of the components are needed for this tutorial.
Notice that the download packace includes a version of the jQuery library.
Step 2 - The page
I've made just a simple html page with two inputs to demonstrate our color chooser.
<?xml version="1.0" encoding="ISO-8859-1" ?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1" />
<link type="text/css" href="css/ui-darkness/jquery-ui-1.7.2.custom.css" rel="stylesheet" />
<script src="js/jquery-1.3.2.min.js" type="text/javascript"></script>
<script src="js/jquery-ui-1.7.2.custom.min.js" type="text/javascript"></script>
<script src="js/colorpicker.js" type="text/javascript"></script>
<style type="text/css">
#red, #green, #blue {
float: left;
clear: left;
width: 300px;
margin: 15px;
}
#swatch {
width: 120px;
height: 100px;
margin-top: 18px;
margin-left: 350px;
background-image: none;
}
#red .ui-slider-range { background: #ef2929; }
#red .ui-slider-handle { border-color: #ef2929; }
#green .ui-slider-range { background: #8ae234; }
#green .ui-slider-handle { border-color: #8ae234; }
#blue .ui-slider-range { background: #729fcf; }
#blue .ui-slider-handle { border-color: #729fcf; }
</style>
<title>Color picker demo</title>
</head>
<body>
<h1>Set the colors</h1>
Color #1: <input type="text" name="color_1" id="color_1" value="" /><br />
Color #2: <input type="text" name="color_2" id="color_2" value="" /><br />
<div id="colorpicker" title="Choose a color">
<div id="red"></div>
<div id="green"></div>
<div id="blue"></div>
<div id="swatch" class="ui-widget-content ui-corner-all"></div>
<input type="hidden" name="selector" id="selector" />
</div>
</body>
</html>
I've included the theme's style sheet (notice that depending on the theme you choosed the path will vary), the jQuery library, the jQueryUI library and our own java script file.
The div colorpicker will be our dialog and the div's red, green and blue will be our sliders.
The jQueryUI makes adding these components into an html page a breeze.
Step 3 - Javascript
Make a new javascript file in the js/ folder and name it colorpicker.js.
If you open the jQueryUI page and look through the demos, you'll see the color chooser demo. Let's add those functions into our js file.
function hexFromRGB (r, g, b) {
var hex = [
r.toString(16),
g.toString(16),
b.toString(16)
];
$.each(hex, function (nr, val) {
if (val.length == 1) {
hex[nr] = '0' + val;
}
});
return hex.join('').toUpperCase();
}
function refreshSwatch() {
var red = $("#red").slider("value")
,green = $("#green").slider("value")
,blue = $("#blue").slider("value")
,hex = hexFromRGB(red, green, blue);
$("#swatch").css("background-color", "#" + hex);
}
$(function() {
$("#red, #green, #blue").slider({
orientation: 'horizontal',
range: "min",
max: 255,
value: 127,
slide: refreshSwatch,
change: refreshSwatch
});
$("#red").slider("value", 255);
$("#green").slider("value", 140);
$("#blue").slider("value", 60);
});
That code will create for us the silders and handle the conversion routine between the slider values and the hex color value.
Add an automaticly runned function with the following markup.
$(function() {
});
What ever is inside this function will be executed when the file is loaded. Inside it, let's add our dialog function.
$('input').click(function() {
$('#selector').val(this.id);
$('#colorpicker').dialog('open');
});
Now when the imputs are clicked, we'll store their id into the hidden selector field to know into which input we need to return the color value.
Then we simply open the color chooser dialog. But as we have not yet created that dialog we must add also the following lines.
$("#colorpicker").dialog({
bgiframe: true,
autoOpen: false,
width: 600,
height: 350,
modal: true,
buttons: {
'Choose a color': function() {
var elem = $("#selector").val();
var color = $("#swatch").css("background-color");
$("#" + elem).val(color);
$(this).dialog('close');
},
Cancel: function() {
$(this).dialog('close');
}
},
close: function() { }
});
This instructs the jQueryUI library to create the kind of dialog we want and to add the buttons into it.
When the Choose a color button is clicked we get the color of the swatch element and return it into the input that was clicked.
Then we just close dialog and let the user continue their surfing.
Conclusions
This has been a short tutorial on how to take the jQueryUI color chooser demo one step further and turn it into a useable color chooset dialog widget.
Hope you have enjoyed reading and learnt something new. Thaks for reading!